The Cancellation Component
Description
Description
This component is used by end users to cancel their travel order online.
It does so by first displaying a form (Screenshot Step 1
) where the user needs to enter the following:
- First Name
- Last Name
- Email Address
- Booking Code
If a booking for the given Booking Code is found the names and email address are checked to authenticate the user. In case of a problem the user is shown a generic error message.
If successful, the travel details and cancellation conditions are displayed (Screenshot Step 2
).
The user can then choose to cancel the order which must be confirmed in a dialog (Screenshot Step 3
).
Afterwards the cancellation will be performed and depending on the success either a Confirmation Page is shown (Screenshot Step 4
) or an Error Page (Screenshot Step 5
).
Technical Overview
To be flexible in how it is integrated in the page and where relevant help text is shown this component is split into multiple parts which are wired together with a script.
Integration
Embed the scripts and styles
Add the following lines to the <head>
section of your site:
<script src="https://api.tui.com/ml/cotton-ball/"></script>
<script type="module" src="https://cloud.tui.com/common/ui-elements/master/ui-elements/ui-elements.esm.js" async="async" defer="defer"></script>
<script nomodule src="https://cloud.tui.com/common/ui-elements/master/ui-elements/ui-elements.js"></script>
<link rel="stylesheet" href="https://cloud.tui.com/common/ui-elements/master/ui-elements/ui-elements.css">
<script type="module" src="https://cloud.tui.com/cdn/cancellation/current/cancellation.esm.js" async="async" defer="defer"></script>
<script nomodule src="https://cloud.tui.com/cdn/cancellation/current/cancellation.js"></script>
Embed the web component
Add the following code in the <body>
section on each page of your website:
<tui-cancellation variant="self-service" tenant="tuicom" locale="de-DE"></tui-cancellation>
<tui-offer-card locale="de-DE">
<tui-cancellation-confirmation locale="de-DE"></tui-cancellation-confirmation>
</tui-offer-card>
<tui-cancellation-result locale="de-DE"></tui-cancellation-result>
Choose a matching tenant and locale.
Wiring Script Example
Add the following script section in your HTML source in order to wire the components together.
This example uses ui-modal
which is part of ui-elements
to show the confirmation dialog.
<script>
// show link to modal in hotel-offer-card
document.querySelector('tui-cancellation').addEventListener('cancellation.showInformation', e => {
document.querySelector('tui-cancellation-confirmation').dispatchEvent(new CustomEvent('cancellation.handleInformation', { detail: e.detail }));
});
// creating and showing modal
document.querySelector('tui-offer-card').addEventListener('cancellation.showConfirmation', e => {
const tuiCancellationElement = document.querySelector('tui-cancellation');
const uiModal = document.createElement('ui-modal');
uiModal.innerHTML = e.detail;
tuiCancellationElement.shadowRoot.appendChild(uiModal);
// closing or aborting modal
tuiCancellationElement.addEventListener('cancellation.closeConfirmation', e => {
uiModal.remove();
_removeListeners()
});
uiModal.addEventListener('clickedInactiveSpace', e => {
uiModal.remove();
_removeListeners()
});
uiModal.addEventListener('modalClosed', _removeListeners);
tuiCancellationElement.addEventListener('cancellation.cancelSuccess', _cancelSuccess);
tuiCancellationElement.addEventListener('cancellation.cancelError', _cancelError);
function _cancelSuccess() {
uiModal.remove();
// hide components
tuiCancellationElement.style.display = 'none'
document.querySelector('tui-offer-card').style.display = 'none'
// show result page
const tuiCancellationResultElement = document.querySelector('tui-cancellation-result')
tuiCancellationResultElement.setAttribute('is-successful', true)
tuiCancellationResultElement.setAttribute('is-show-result', true)
}
function _cancelError() {
uiModal.remove();
// hide components
tuiCancellationElement.style.display = 'none'
document.querySelector('tui-offer-card').style.display = 'none'
// show result page
const tuiCancellationResultElement = document.querySelector('tui-cancellation-result')
tuiCancellationResultElement.setAttribute('is-successful', false)
tuiCancellationResultElement.setAttribute('is-show-result', true)
}
function _removeListeners() {
tuiCancellationElement.removeEventListener('cancellation.cancelSuccess', _cancelSuccess);
tuiCancellationElement.removeEventListener('cancellation.cancelError', _cancelSuccess);
}
});
</script>
Error Page Link
The error display in Screenshot Step 5
contains a button which by default links to https://www.tui.com/tui-info-corona-virus/?contentid=2342_kachelmenue_umbu_20210609#c90052
This can be overwritten by setting info-url on the tui-cancellation-result tag:
<tui-cancellation-result info-url="https://example.com" locale="de-DE"></tui-cancellation-result>
Styling
The component can be styled by adding a custom attribute brand
to the body or anywhere in the DOM above the component:
<body brand="tui">
Available Brands:
tui
airtours
robinson
ltur
tuimagiclife
gulet
Screenshots
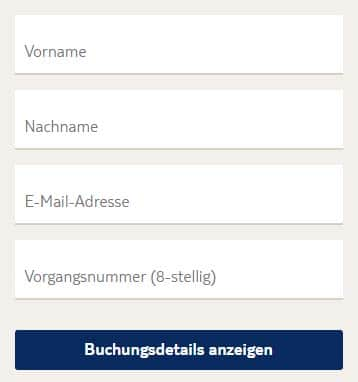
Step 1: Form where the user enters their order details
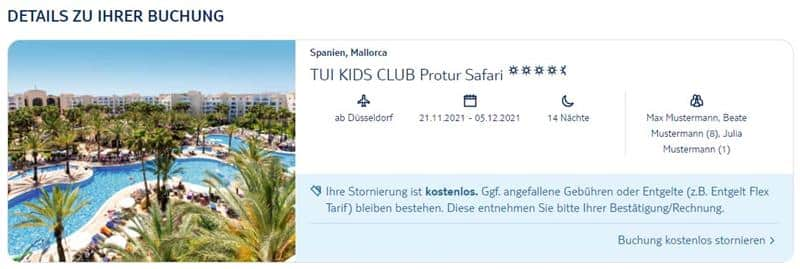
Step 2: Travel details and cancellation conditions are displayed
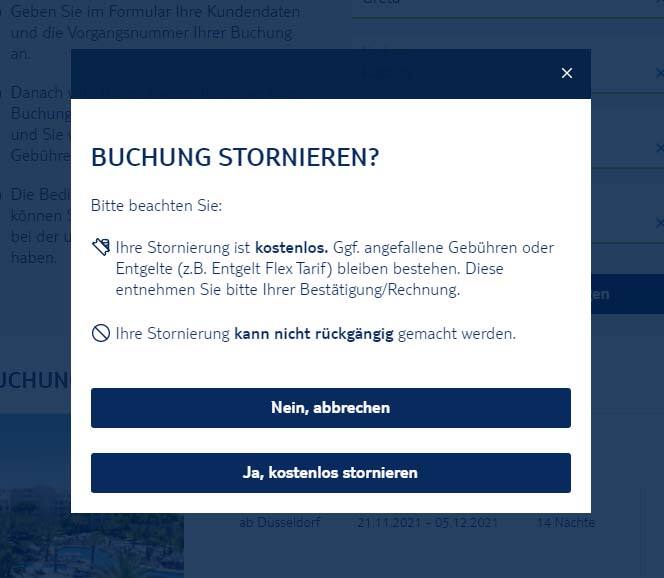
Step 3: Modal asks the user for confirmation
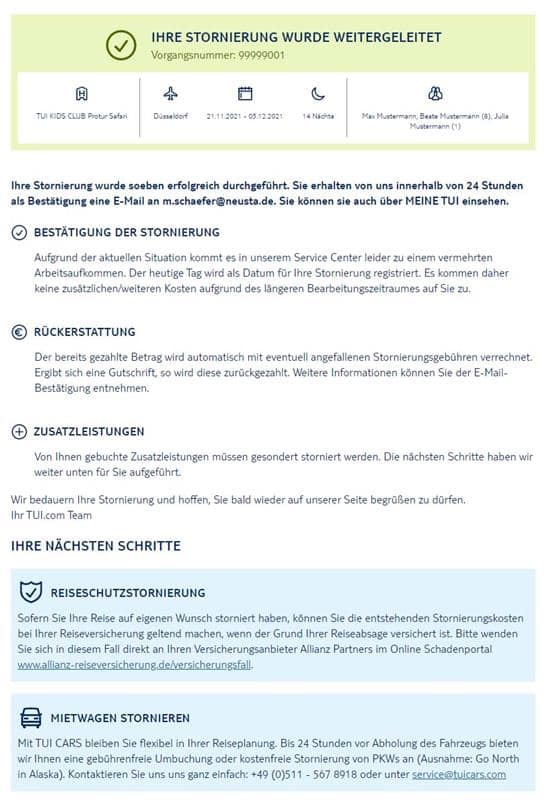
Step 4: confirmation page is presented on success
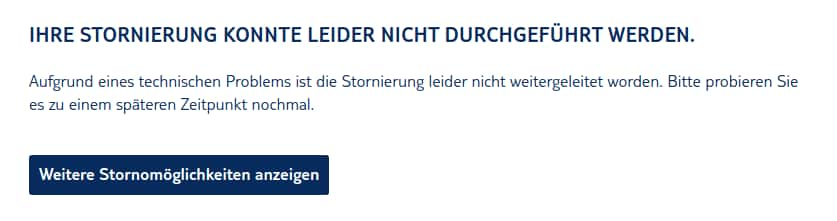
Step 5: error page is presented on error
Meta
Maintainer | mlist-tuicom-neusta-op@neusta.de |
---|---|
Locales |
fr-CH , de-DE
|
Usage
Add the following script tag (or something similar) to your web page to have the component load. Note: This is required only once per webpage, even when you embed multiple instances of the component.
<script src="https://cloud.tui.com/cdn/cancellation/current/cancellation.esm.js" async defer></script>
Add the following HTML code anywhere in your webpage at the position where you want the component to show. You can create multiple instances of the component.
Remember to replace VALUE
with actual valid values for the given attribute.
<tui-cancellation variant="VALUE"></tui-cancellation>
Examples
-
Simple embedding of the component, preconfigured with tui.com links.
<tui-cancellation variant="self-service" tenant="tuicom" locale="de-DE"> </tui-cancellation>
Read more about using components ›
Attributes
This component can be configured with the following attributes:
Name | Description | Allowed Values | Default | Required |
---|---|---|---|---|
tenant |
Tenant to use for Backend calls |
tuicom , tuiat , tuich , lturde , lturat , lturch , ati , robinson , magiclife , tuiblue
|
tuicom |
no |
agency |
Optionally overwrites agency number used for BookApi calls |
no | ||
variant |
Cancellation variant to use |
self-service
|
yes |
Events
Event | cancellation.showInformation |
||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Type | publish | ||||||||||||||
Description | Publishes the cancellation conditions. Published after the user enters their data and it is successfully authenticated. |
||||||||||||||
Payload |
The payload object of this event has the following properties:
|
Event | cancellation.cancelSuccess |
|||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Type | publish | |||||||||||||||||||||
Description | Publishes the cancellation result. Published after the user confirms they want to cancel and it is successfully cancelled. |
|||||||||||||||||||||
Payload |
The payload object of this event has the following properties:
|
Event | cancellation.cancelError |
|||||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
Type | publish | |||||||||||||||||||||
Description | Publishes the cancellation result. Published after the user confirms they want to cancel and it failed to be cancelled. |
|||||||||||||||||||||
Payload |
The payload object of this event has the following properties:
|